ポリラインの勾配を計算する。傾きと角度それぞれをアトリビュートに追加している。
ポイントの勾配を計算
//
// 縦断方向の勾配と角度を計算する
// Run Over: Primitives
//
int pts[] = primpoints(0, @primnum);
for(int i = 1; i < len(pts); i++)
{
vector p0 = point(0, "P", pts[i-1]);
vector p1 = point(0, "P", pts[i]);
vector diff = p1 - p0;
float h = diff.y;
diff.y = 0;
float bottom = length(diff);
// 勾配の計算
float slope = h / bottom;
setpointattrib(0, "__slope", pts[i], slope);
// 角度(ラジアン)の計算
float theta = atan2(h, bottom);
setpointattrib(0, "__angle", pts[i], theta);
}
ポリラインの平均勾配を計算
//
// 縦断方向の平均勾配と角度を計算する
// Run Over: Primitives
//
int pts[] = primpoints(0, @primnum);
float sum_y = 0;
float sum_x = 0;
for(int i = 1; i < len(pts); i++)
{
vector p0 = point(0, "P", pts[i-1]);
vector p1 = point(0, "P", pts[i]);
vector diff = p1 - p0;
float h = diff.y;
diff.y = 0;
float bottom = length(diff);
sum_y += h;
sum_x += bottom;
}
// 勾配の計算
float slope = sum_y / sum_x;
slope = abs(slope) * 100;
setprimattrib(0, "__slope", @primnum, slope);
// 角度(ラジアン)の計算
float theta = atan2(sum_y, sum_x);
setprimattrib(0, "__angle", @primnum, theta);
視覚化
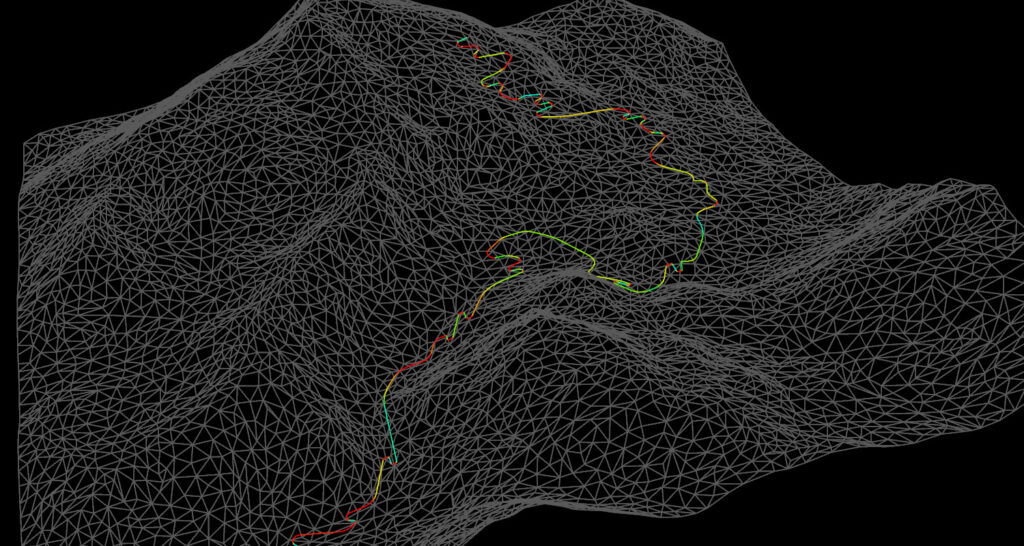
//
// 縦断方向の勾配を計算し、色をつける
// Run Over: Primitives
//
float maxValue = chf("slope"); // 上限の勾配(%)(赤く表示される値)
int pts[] = primpoints(0, @primnum);
for(int i = 1; i < len(pts); i++)
{
vector p0 = point(0, "P", pts[i-1]);
vector p1 = point(0, "P", pts[i]);
vector diff = p1 - p0;
float h = diff.y;
diff.y = 0;
float bottom = length(diff);
// 勾配の計算(傾き、100倍して%の単位に合わせる)
float slope = h / bottom * 100;
setpointattrib(0, "__slope", pts[i], slope);
float value = abs(slope);
float hue = clamp(value/maxValue, 0, 1.0) * 0.5 * -1 + 0.5;
vector color = hsvtorgb(hue, 1, 0.8);
setpointattrib(0, "Cd", pts[i], color);
}