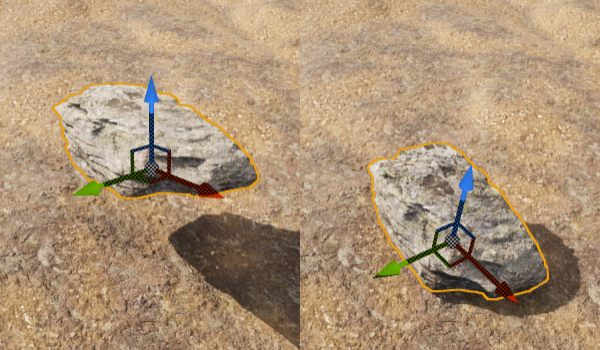
レイを飛ばしてスタティックメッシュとの交差座標を取得する。
#
# スタティックメッシュとの交差点を計算する
#
import unreal
selectedActors = unreal.EditorLevelLibrary.get_selected_level_actors()
if len(selectedActors) == 1:
for actor in selectedActors:
primitiveComponent = actor.get_component_by_class(unreal.PrimitiveComponent)
print(primitiveComponent)
start = unreal.Vector(0, 0, 100000)
end = unreal.Vector(0, 0, -100000)
result = primitiveComponent.line_trace_component(start, end, False, False, False)
if(result is not None):
print("pos:" + str(result[0]))
print("normal:" + str(result[1]))
primitiveComponent.line_trace_component で特定のメッシュの交差座標を計算する。
コリジョンメッシュに対して選択アクターを接地させる
#
# コリジョンメッシュに接地させる
#
import unreal
# コリジョンメッシュ
actor_name = 'PersistentLevel.SM_Mountain_2'
targetActor = unreal.EditorLevelLibrary.get_actor_reference(actor_name)
print(targetActor)
# 選択メッシュ
selectedActors = unreal.EditorLevelLibrary.get_selected_level_actors()
if targetActor is not None and len(selectedActors) > 0:
for actor in selectedActors:
primitiveComponent = targetActor.get_component_by_class(unreal.PrimitiveComponent)
print(primitiveComponent)
#transform = actor.get_actor_transform()
location = actor.get_actor_location()
start = location
end = location + unreal.Vector(0, 0, -100000)
# 交差座標
result = primitiveComponent.line_trace_component(start, end, True, False, False)
if(result is not None):
pos = result[0]
# 座標
actor.set_actor_location(pos, False, False)
# 回転の計算
# フォワードベクター(X軸)を取得する
forwardVec = actor.get_actor_forward_vector()
# ライトベクター(Y軸)を取得する
rightVec = actor.get_actor_right_vector()
# アップベクター(Z軸)を取得する
upVec = actor.get_actor_up_vector()
normal = result[1]
forwardVec = unreal.Vector.cross(rightVec, normal)
# 座標変換(行列から回転を生成する)
matrix = unreal.Matrix([forwardVec.x,forwardVec.y,forwardVec.z,0], [rightVec.x,rightVec.y,rightVec.z,0], [upVec.x,upVec.y,upVec.z,0], [0,0,0,0])
rotation = matrix.get_rotator()
actor.set_actor_rotation(rotation, False)
アクターを指定してコリジョンを取得する方法。
最初に交差するオブジェクトを探して接地する
#
# コリジョンメッシュに接地させる
#
import unreal
# 選択メッシュ
selectedActors = unreal.EditorLevelLibrary.get_selected_level_actors()
if len(selectedActors) > 0:
for actor in selectedActors:
location = actor.get_actor_location()
# 真下の場合
start = location
end = location + unreal.Vector(0, 0, -100000)
# アクターのダウンベクターの場合
#upVec = actor.get_actor_up_vector()
#start = location
#end = location + upVec * -100000
# 交差判定
# https://docs.unrealengine.com/4.26/en-US/PythonAPI/class/SystemLibrary.html#unreal.SystemLibrary.line_trace_single
actors_ignore = []
hitResult = unreal.SystemLibrary().line_trace_single(
unreal.EditorLevelLibrary().get_editor_world(),
start,
end,
unreal.TraceTypeQuery.TRACE_TYPE_QUERY1,
True,
actors_ignore,
unreal.DrawDebugTrace.NONE,
True)
if(hitResult is not None):
# 座標
pos = hitResult.to_tuple()[4]
actor.set_actor_location(pos, False, False)
# 回転
normal = hitResult.to_tuple()[6]
# フォワードベクター(X軸)を取得する
forwardVec = actor.get_actor_forward_vector()
# ライトベクター(Y軸)を取得する
rightVec = actor.get_actor_right_vector()
# アップベクター(Z軸)を取得する
upVec = actor.get_actor_up_vector()
forwardVec = unreal.Vector.cross(rightVec, normal)
# 座標変換(行列から回転を生成する)
matrix = unreal.Matrix([forwardVec.x,forwardVec.y,forwardVec.z,0], [rightVec.x,rightVec.y,rightVec.z,0], [upVec.x,upVec.y,upVec.z,0], [0,0,0,0])
rotation = matrix.get_rotator()
actor.set_actor_rotation(rotation, False)
unreal.EditorLevelLibrary.set_selected_level_actors(selectedActors)
unreal.SystemLibrary().line_trace_single
これならランドスケープとの交差座標も計算できる。
unreal.HitResult
//https://docs.unrealengine.com/4.26/en-US/PythonAPI/class/HitResult.html
ランドスケープのみに接地させる
#
# ランドスケープに接地させる
#
import unreal
# 選択メッシュ
selectedActors = unreal.EditorLevelLibrary.get_selected_level_actors()
if len(selectedActors) > 0:
for actor in selectedActors:
location = actor.get_actor_location()
# 真下の場合
start = location
end = location + unreal.Vector(0, 0, -100000)
# アクターのダウンベクターの場合
#upVec = actor.get_actor_up_vector()
#start = location
#end = location + upVec * -100000
# 交差判定
# https://docs.unrealengine.com/4.26/en-US/PythonAPI/class/SystemLibrary.html#unreal.SystemLibrary.line_trace_single
actors_ignore = []
hitResults = unreal.SystemLibrary().line_trace_multi(
unreal.EditorLevelLibrary().get_editor_world(),
start,
end,
unreal.TraceTypeQuery.TRACE_TYPE_QUERY1,
True,
actors_ignore,
unreal.DrawDebugTrace.NONE,
True)
if(hitResults is not None):
for result in hitResults:
if result.to_tuple()[9].get_class().get_name() == 'Landscape':
# 座標
pos = result.to_tuple()[4]
actor.set_actor_location(pos, False, False)
# 回転
normal = result.to_tuple()[6]
# フォワードベクター(X軸)を取得する
forwardVec = actor.get_actor_forward_vector()
# ライトベクター(Y軸)を取得する
rightVec = actor.get_actor_right_vector()
# アップベクター(Z軸)を取得する
upVec = actor.get_actor_up_vector()
forwardVec = unreal.Vector.cross(rightVec, normal)
# 座標変換(行列から回転を生成する)
matrix = unreal.Matrix([forwardVec.x,forwardVec.y,forwardVec.z,0], [rightVec.x,rightVec.y,rightVec.z,0], [upVec.x,upVec.y,upVec.z,0], [0,0,0,0])
rotation = matrix.get_rotator()
actor.set_actor_rotation(rotation, False)
unreal.EditorLevelLibrary.set_selected_level_actors(selectedActors)