フラットシェーディングのようなローポリ向けのシェーダーをカスタムシェーダで書く。
頂点カラーを使う
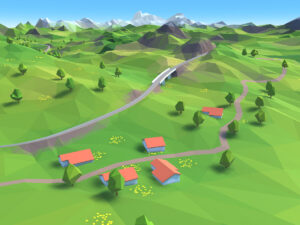
Standard Surface Shaderをつくり、数行書き加える。
Shader "Custom/LowpolySurfaceShader"
{
Properties
{
_Color ("Color", Color) = (1,1,1,1)
_MainTex ("Albedo (RGB)", 2D) = "white" {}
_Glossiness ("Smoothness", Range(0,1)) = 0.5
_Metallic ("Metallic", Range(0,1)) = 0.0
}
SubShader
{
Tags { "RenderType"="Opaque" }
LOD 200
CGPROGRAM
// Physically based Standard lighting model, and enable shadows on all light types
#pragma surface surf Standard fullforwardshadows vertex:vert
// Use shader model 3.0 target, to get nicer looking lighting
#pragma target 3.0
sampler2D _MainTex;
struct Input
{
float2 uv_MainTex;
float4 vertexColor;
};
void vert(inout appdata_full v, out Input o)
{
UNITY_INITIALIZE_OUTPUT(Input, o);
o.vertexColor = v.color;
}
half _Glossiness;
half _Metallic;
fixed4 _Color;
// Add instancing support for this shader. You need to check 'Enable Instancing' on materials that use the shader.
// See https://docs.unity3d.com/Manual/GPUInstancing.html for more information about instancing.
// #pragma instancing_options assumeuniformscaling
UNITY_INSTANCING_BUFFER_START(Props)
// put more per-instance properties here
UNITY_INSTANCING_BUFFER_END(Props)
void surf (Input IN, inout SurfaceOutputStandard o)
{
// Albedo comes from a texture tinted by color
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
//o.Albedo = c.rgb;
o.Albedo = c.rgb * IN.vertexColor;
// Metallic and smoothness come from slider variables
o.Metallic = _Metallic;
o.Smoothness = _Glossiness;
o.Alpha = c.a;
}
ENDCG
}
FallBack "Diffuse"
}
エミッシブを使う
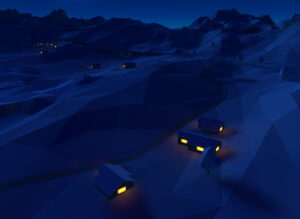
グローバルイルミネーションのベイクのためにメタパスを使う。
Shader "Custom/LowpolyEmission"
{
Properties
{
_Color ("Color", Color) = (1,1,1,1)
_MainTex ("Albedo (RGB)", 2D) = "white" {}
_Glossiness ("Smoothness", Range(0,1)) = 0.5
_Metallic ("Metallic", Range(0,1)) = 0.0
_EmissionColor("Emission Color", Color) = (1.0, 1.0, 1.0, 1.0)
_EmissionTex("Emission Texture", 2D) = "White" {}
_EmissionIntensity("Emission Intensity", Range(0,16)) = 1.0
_GIAlbedoColor ("Color Albedo (GI)", Color)=(1,1,1,1)
_GIAlbedoTex ("Albedo (GI)",2D)="white"{}
}
SubShader
{
pass
{
Name "META"
Tags {"LightMode" = "Meta"}
Cull Off
CGPROGRAM
#include"UnityStandardMeta.cginc"
#pragma vertex vert_meta
#pragma fragment frag_meta_custom
#pragma shader_feature _EMISSION
#pragma shader_feature _METALLICGLOSSMAP
#pragma shader_feature ___ _DETAIL_MULX2
#pragma shader_feature EDITOR_VISUALIZATION
sampler2D _GIAlbedoTex;
fixed4 _GIAlbedoColor;
float4 frag_meta_custom (v2f_meta i): SV_Target
{
FragmentCommonData data = UNITY_SETUP_BRDF_INPUT (i.uv);
UnityMetaInput o;
UNITY_INITIALIZE_OUTPUT(UnityMetaInput, o);
fixed4 c = tex2D (_MainTex, i.uv);
o.Albedo = fixed3(c.rgb * _GIAlbedoColor.rgb);
//o.SpecularColor = float3(1, 0, 0);
o.Emission = _EmissionColor;
return UnityMetaFragment(o);
}
ENDCG
}
Tags { "RenderType"="Opaque"}
LOD 200
CGPROGRAM
// Physically based Standard lighting model, and enable shadows on all light types
#pragma surface surf Standard fullforwardshadows vertex:vert
// Use shader model 3.0 target, to get nicer looking lighting
#pragma target 3.0
sampler2D _MainTex;
sampler2D _EmissionTex;
half4 _EmissionColor;
float _EmissionIntensity;
half _Glossiness;
half _Metallic;
fixed4 _Color;
struct Input
{
float2 uv_MainTex;
float4 vertexColor;
};
void vert(inout appdata_full v, out Input o)
{
UNITY_INITIALIZE_OUTPUT(Input, o);
o.vertexColor = v.color;
}
// Add instancing support for this shader. You need to check 'Enable Instancing' on materials that use the shader.
// See https://docs.unity3d.com/Manual/GPUInstancing.html for more information about instancing.
// #pragma instancing_options assumeuniformscaling
UNITY_INSTANCING_BUFFER_START(Props)
// put more per-instance properties here
UNITY_INSTANCING_BUFFER_END(Props)
void surf (Input IN, inout SurfaceOutputStandard o)
{
// Albedo comes from a texture tinted by color
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
//o.Albedo = c.rgb;
float e = tex2D(_EmissionTex, IN.uv_MainTex).r;
o.Albedo = c.rgb * IN.vertexColor;
// Metallic and smoothness come from slider variables
o.Metallic = _Metallic;
o.Smoothness = _Glossiness;
o.Alpha = c.a;
o.Emission = e * _EmissionColor * _EmissionIntensity;
}
ENDCG
}
FallBack "Diffuse"
}
グローバルイルミネーションを有効にするためにはC#スクリプトをGameオブジェクトにアタッチする必要がある。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GlobalIlluminationFlags : MonoBehaviour
{
public MaterialGlobalIlluminationFlags flag = MaterialGlobalIlluminationFlags.AnyEmissive;
void OnValidate()
{
var renderer = GetComponent<Renderer>();
if(renderer && renderer.sharedMaterial)
{
renderer.sharedMaterial.globalIlluminationFlags = flag;
}
}
}